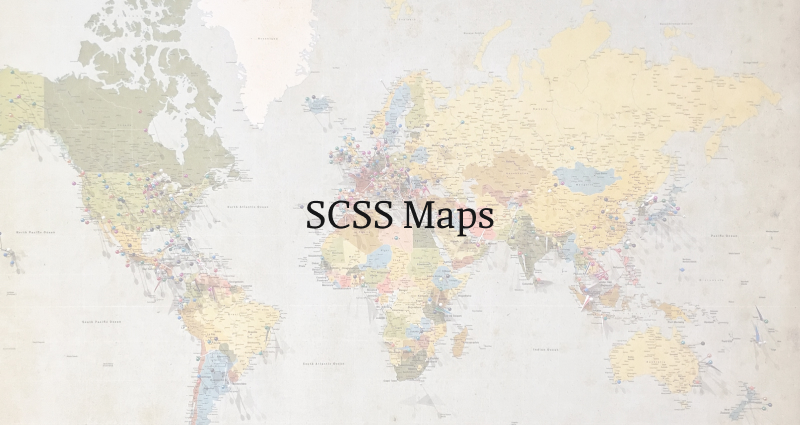
A lot of front end developers are using SCSS these days. It is a powerful extension to CSS. With it, you get the power of variables, nesting, partials, imports, mixins, inheritance, operators and more. But one of the lesser known features is known as maps. This feature has been around for a long time, yet it is mostly unknown or at the very least, underused.
Maps Basics
SCSS maps are basically an associative arrays or hashes in other languages. In other words, it is an array with matching keys to values.
Lets look at the syntax:
$map: (
key: value,
key2: value2
);
Some things to keep in mind. Keys must be unique. Keys and values can be any SCSS type including other maps.
Getting Values from Map
In order to get a value from a map you use the map-get function.
Syntax:
map-get($map, $key);
In practice
So what are maps good for? Anything you want really. A basic case is defining colors for a project.
$colors: (
primary: tomato,
secondary: slateblue
);
h1 {
color: map-get($colors, primary);
}
Output:
h1 {
color: tomato;
}
Taking it a Step Further
Let us set up a way to make break points much easier. Let's set up our map first.
$breakpoints: (
desktop: 1100px,
tablet: 768px,
mobile: 480px
);
Now let's set up a mixin to automatically output what we need for the breakpoint.
@mixin responsive($breakpoint) {
@if map-has-key($breakpoints, $breakpoint) {
@media (max-width: #{map-get($breakpoints, $breakpoint)}) {
@content
}
}
@else {
@warn "No breakpoint has been found";
}
}
Usage
h1 {
color: tomato;
@include responsive(tablet) {
color: slateblue;
}
}
Output
h1 {
color: tomato;
}
@media (max-width: 768px) {
h1 {
color: slateblue;
}
}
Setting up Color Themes with Maps
One of the coolest ways to use maps is setting up themes. Say you have a website with portfolio items. If you want each one themed differently you can specify different colors for each one.
Let's set up a few right now using maps.
$themes: (
blue: (
color: #33A5FF,
background: #CDE9FF,
),
yellow: (
color: #FDFFA4,
background: #E8EC00,
),
red: (
color: #FEBFC6,
background: #F10622,
),
purple: (
color: #FEBFF5,
background: #AD0BDA,
)
);
As you can see we have a multi level map here. Lets create a mixin that will automatically output a property of our choosing with the color we need. It will be basic, so if you need to add more complexity, feel free.
@mixin theme($property, $value) {
@each $theme, $map in $themes {
.theme-#{$theme} & {
#{$property}: map-get($map, $value);
}
}
}
Usage:
h1 {
@include theme(color, background);
}
Output
.theme-blue h1 {
color: #CDE9FF;
}
.theme-yellow h1 {
color: #E8EC00;
}
.theme-red h1 {
color: #F10622;
}
.theme-purple h1 {
color: #AD0BDA;
}
Conclusion
Maps are a really powerful way to add functionality and organization to your CSS. There are a lot of more things you can do with them. Have a look at the available functions available at https://sass-lang.com/documentation/functions/map