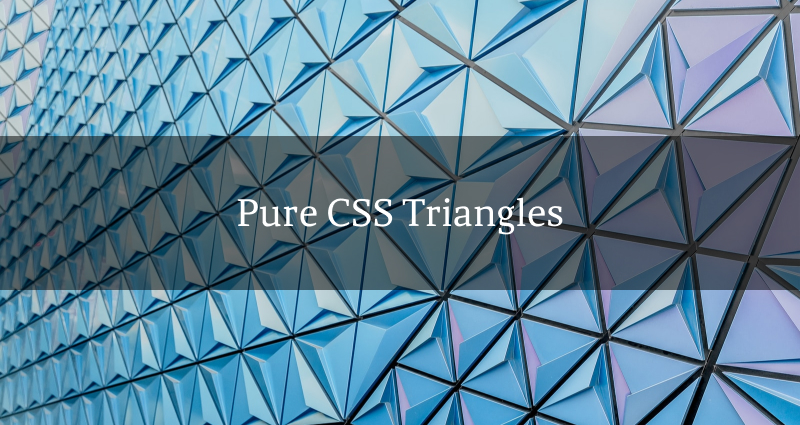
Ever since I redid my website a often requested tutorial is from those looking how to do pure CSS triangles. It was my most visited tutorial and one that showed up well in search rankings. That showed me that people were eager to learn how to do it. I am happy to redo the tutorial so people can read it again years later. So without further adieu read on to learn how to do pure CSS triangles.
The first thing to learn is how exactly triangles are formed with pure CSS. There is no confusing math or SVG number wizardry (though certainly you can do that). To learn the base of a CSS triangles you have to learn how CSS borders are made by browsers. If we create an object that has no height, no width. and apply a border of different colors to each side we find something quite amazing.
For example take the following code
.triangle-basic {
height: 0;
width: 0;
border: 30px solid;
border-top-color: red;
border-right-color: blue;
border-bottom-color: green;
border-left-color: orange;
}
Pretty simple. If you are used to CSS, nothing here should be new. Now lets look at what this looks like in the browser.

See that? Triangles. Did something just click for you?
So how do we isolate just one? Pretty simple actually. Take a look at the next example
.triangle-simple {
height: 0;
width: 0;
border-left: 30px solid red;
border-top: 30px solid transparent;
border-bottom: 30px solid transparent;
}
Take a second to see what is going on. We are defining one section of the border (the visible triangle), and the two neighbor sides get the same treatment minus the color which is transparent. This is done for the triangle’s height integrity.

That is the basics of your standard pure CSS triangle.
Let’s take things up a notch. Let’s make a pure CSS arrow. It is done in two parts: the triangle (tip of the arrow) and the base.
The base is simply a box which is pretty standard minus one additions I will go over.
.triangle-arrow {
width: 100px;
height: 50px;
background: #ccc;
position: relative;
}
Whats’s new here is the addition of position:relative;. This is so we can absolute position the tip of the arrow. Now to do the tip we are going to use the pseudo class :after.
.triangle-arrow:after {
content: '';
position: absolute;
top: -25px;
right: -50px;
border-left: 50px solid #ccc;
border-top: 50px solid transparent;
border-bottom: 50px solid transparent;
}
We create the triangle with a 50px value (in this case it is width since it is a left border) and then just absolute position it.
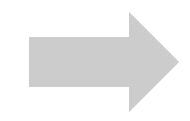
Now for a real world example. Creating a button that takes advantage of a CSS triangle. Let’s get some basic markup.
<div class="triangle-advanced">
<a href="#">Advanced Button</a>
</div>
The class can be just applied to the link itself but for demo purposes I did it like this.
Now for the basic button style.
.triangle-advanced a {
padding: 15px;
padding-right: 45px;
background: #5A9FD2;
color: #fff;
text-decoration: none;
display: inline-block;
font-size: 13px;
position: relative;
border-radius: 20px;
}
You will notice that I left a big padding on the right. this is going to make room for our arrow. We are going to be doing the arrow in two parts. Our design calls for the arrow to have a darker blue circle around it. We are going to be using both :before and :after and then absolute position each. If you have read the previous parts to this tutorial you should already have an idea how this is going to work.
First the circle:
.triangle-advanced a:before {
content: '';
position: absolute;
top: 8px;
right: 10px;
width: 25px;
height: 25px;
border-radius: 50%;
background: #4A83AD;
}
And now for the arrow:
.triangle-advanced a:after {
content: '';
position: absolute;
top: 15px;
right: 18px;
height: 0;
width: 0;
border-left: 6px solid #5A9FD2;
border-top: 6px solid transparent;
border-bottom: 6px solid transparent;
}
You can also apply hover states to them as well, but we won’t be going over that in this tutorial.
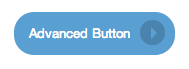
As for browser support. You can do CSS triangles in IE7+ as well as modern browsers. IE6 technically supports it, but it doesn’t recognize the color transparent. There are some fixes for that, but I think pretty much everyone has dropped support for IE6 at this point. The pseudo class are supported in IE8+ as well as modern browsers.
See the Pen shpBb by Christopher Bishop (@cibgraphics) on CodePen.
Enjoy.