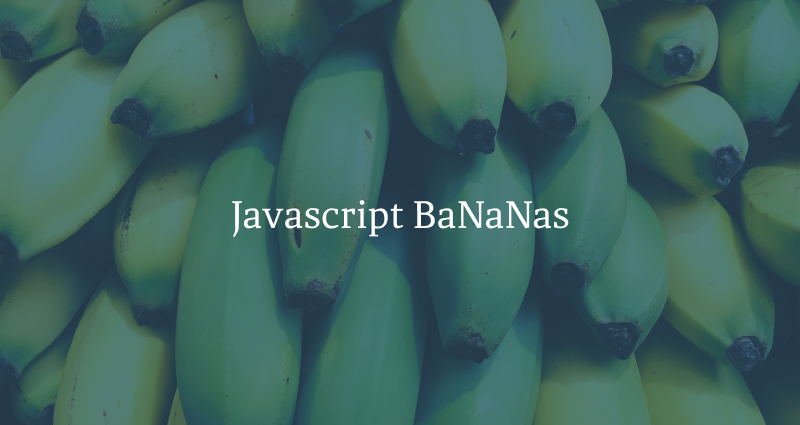
Javascript is weird. Like really weird. This article is showing a stupid party trick with Javascript, but at the same time explaining why it does what it does.
The code in question is
document.write(('b' + 'a' + + 'a' + 'a').toLowerCase());
This outputs “banana”. But why?
Dynamic Types
To start learning why, we need to learn how Javascript treats data types. Unlike a lot of other languages, Javascript has what is called dynamic typing. This means that variables are not directly associated with a particular value type. For example var foo = 42, foo would be a number type. Reassigning the same variable foo = ‘bar’, foo is now a string. You don’t need to explicitly state that the new variable content is now a string. It handles it automatically.
Unary Operators
A basic operator in Javascript can be as simple as 1+1 (which outputs 2). The operator in this example is the +, and both 1’s are considered operands. A unary operator is an operation with only one operand. It come either before or after the operand.
So what does this have to do with bananas?
In the banana example we have a bunch of operators and operands. In the middle we have a very odd ++”a”. The section with just +”a” acts as a unary plus which will convert a string of numbers into a number data type. This acts as a unary plus operator because of the double ++. Since the “a” is not a number, it outputs NaN which stands for “Not A Number”. The first + which is not part of the unary operator adds the “NaN” to the string. Remember that in Javascript, if you add strings together it concatenates them together.
Our string now reads “baNaNa”. After running a simple toLowerCase function on it, we have our final output: banana.
It is a weird example that people love to use to bash Javascript, but it actually makes a lot of sense when you realize what exactly Javascript is doing.