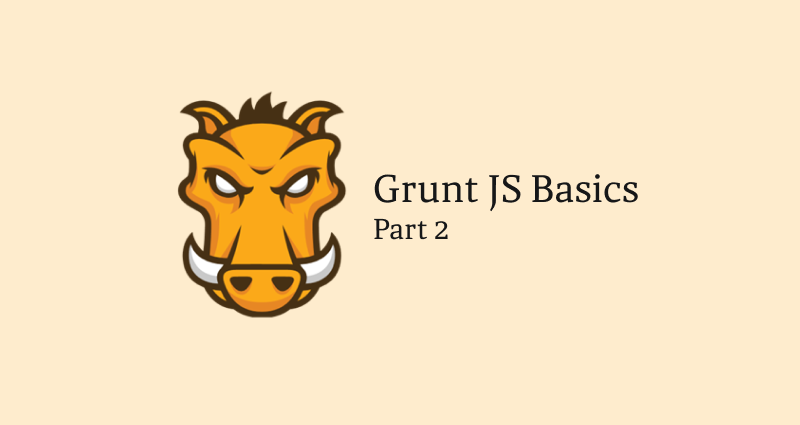
In the last GruntJS article I explained what GruntJS was, how to set it up and use a basic plugin to minify your Javascript. If you have not read that, or don't know what GruntJS is, please read that first. In this article we are going to look at some other plugins such as Watch, using Sass and Neat, and making a SVG sprite file automatically from a folder of SVGs.
Grunt Watch
One of the most useful plugins for GruntJS is the Watch plugin. At the time of this article, in the last 30 days this plugin has been downloaded 1,048,011 times. That is a lot. So what does this plugin do? Simply put, it watches for changes in files and folders and then does something (usually another task). What does that mean? It means that you can have it lint your CSS, compile your Sass, minify your CSS, and reload the browser... all with a single save if a Sass file. Pretty powerful. So lets set it up.
First you will need to install the plugin.
npm install grunt-contrib-watch --save-dev
and of course enable it inside your Gruntfile.js.
grunt.loadNpmTasks('grunt-contrib-watch');
For this example we are just going to reload the browser when we save a HTML file, but it can easily be set up to do CSS, Javascript or anything else.
Inside your Gruntfile.js where you configure your tasks, use:
watch: {
options: {
livereload: true
}
html:{
files: ['**/*.html'],
tasks: [],
options: {
spawn: false
}
}
},
Since we aren't running any tasks when we save an HTML file, we don't put anything in the tasks section. Note that in the options we have set livereload to true. This works with the LiveReload plugin for browsers to refresh the browser when a HTML file is changed.
To be extra helpful, we can even set the Watch plugin to reload itself when we make changes to our Gruntfile.js.
configFiles: {
files: [ 'Gruntfile.js', 'config/*.js' ],
options: {
reload: true
}
}
Setting up SASS with Bourbon Neat
Most front end developers are using SASS. It is a really helpful compiler for CSS. If you are not using it to write your CSS, you should. Bourbon Neat is a SASS based grid framework and honestly my favorite one out of all the ones available.
Lets install a couple plugins.
npm install --save-dev grunt-sass node-neat
If you were paying attention to that line of code, you would notice that we are installing two plugins at once. Helpful trick. This plugin for SASS uses the node version of SASS instead of the Ruby gem version, which will make things much much quicker when compile time comes.
For this we only need to load the SASS plugin because we are going to treat Neat a little differently (you'll see this in a bit).
grunt.loadNpmTasks('grunt-sass');
Lets configure the plugin to do what we want.
sass: {
dist: {
options: {
style: 'nested',
includePaths: require('node-neat').includePaths
},
files: {
'css/style.css': 'css/scss/style.scss',
}
}
},
The paths will be different depending on how you have your project set up, just remember that the path is relative to where the Gruntfile.js file is located. In the options we have an option called includePaths that loads up Neat correctly. To use Neat in your SASS you have a little additional work to do, but it is simple. At the very start of your SASS file just include the following.
@import "bourbon";
@import "neat";
You should be ready to go using Neat inside your SASS now. If you would like to add this to your Watch task, then it is as simple as the HTML one with the exception of running the SASS task as well on watch.
css: {
files: ['css/scss/**/*.scss'],
tasks: ['sass''],
options: {
spawn: false
}
},
SVG Sprite
To some this is a new concept. What is a SVG sprite. If you know what an image sprite is, it is a single image that has a bunch of smaller ones that you can make visible with some CSS. It is much the same concept with SVG sprites. It is a single SVG that has a bunch of other SVG images that you can use on your webpage... but they are vector. SVGStore will make these automatically for you.
npm install grunt-svgstore --save-dev
And enable it.
grunt.loadNpmTasks('grunt-svgstore');
The options for it are a little intense, but read through the documentation for it, and see how you can use it best for your workflow.
svgstore: {
options: {
prefix : 'icon-',
svg: {
viewBox : '0 0 100 100',
xmlns: 'http://www.w3.org/2000/svg',
style: "display: none;"
},
cleanup: ['fill', 'style'],
},
default: {
files: {
'images/svg-sprite.svg' : ['images/svg/*.svg']
}
},
},
The main parts you need to worry about is the prefix, cleanup, and files. The prefix is exactly what it sounds like. It adds a prefix to each svg when you call it in your html. For example, if you have an icon called phone, you use it in your markup as icon-phone if the prefix is like the example above. Cleanup in this case will go through an remove all fill and inline styles in the SVG. It makes it so you start off with a blank slate when using it in your markup. This may or may not be desired so use it as you wish. The files will need to be changed based on your project set up.
Wish to use this as part of your watch as well? Sure thing.
svg: {
files: ['images/svg/*.svg'],
tasks: ['svgstore'],
}
So how do you use a SVG sprite? It is quite easy in fact. First you have to include the SVG right after the opening body tag. I typically use PHP in my files so this is how I would include it in my files. Again, change the paths to what you need.
<?php include_once($_SERVER['DOCUMENT_ROOT']."/images/svg-sprite.svg"); ?>
And then to use a SVG in your markup you the svg and use tags.
<svg>
<use xlink:href="#icon-phone" />
</svg>
You can now style it any way you wish using CSS/SASS.
Finishing up
These are only a couple really useful Grunt plugins to help you get started using GruntJS. There are far too many plugins to write an article about each, but most have really good documentation of what they are and how to use them. Just going through some on the GruntJS Plugin site will give you some really good information on what people are using a lot. So get out there an have fun.